授权流程
三种授权方式
Shiro 支持三种方式的授权:
- 编程式:通过写if/else 授权代码块完成:
1
2
3
4
5
6Subject subject = SecurityUtils.getSubject();
if(subject.hasRole(“admin”)) {
//有权限
} else {
//无权限
}- 注解式:通过在执行的Java方法上放置相应的注解完成:
1
2
3
4@RequiresRoles("admin")
public void hello() {
//有权限
}- JSP/GSP 标签:在JSP/GSP 页面通过相应的标签完成:
1
2
3<shiro:hasRole name="admin">
<!— 有权限—>
</shiro:hasRole>
授权入门程序
进行授权之前首先要进行上一节学习的认证操作,认证通过之后才能进行授权。授权的单元测试代码如下:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51/**
* @Description 测试角色授权,资源授权
* @Author 刘俊重
* @date 2017年8月3日
* @return void
*/
@Test
public void testAuthorization(){
//授权之前需要先进行认证
//创建SecurityManager工厂
Factory<SecurityManager> factory = new IniSecurityManagerFactory("classpath:shiro-permission.ini");
//创建SecurityManager
SecurityManager securityManager = factory.getInstance();
//将SecurityManager设置到当前页面中
SecurityUtils.setSecurityManager(securityManager);
//从SecurityUtils中构建subject(主体)
Subject subject = SecurityUtils.getSubject();
//构建token
UsernamePasswordToken token = new UsernamePasswordToken("zhangsan", "123456");
//提交认证
try {
subject.login(token);
} catch (AuthenticationException e) {
e.printStackTrace();
}
//认证结果
System.out.println("认证结果:"+subject.isAuthenticated());
//认证通过后执行授权
//基于角色的授权,括号内放的是角色
boolean hasRole = subject.hasRole("role1");
System.out.println("单一角色授权:"+hasRole);
//如果没有拥有该角色会抛出异常
//subject.checkRole("role3");
boolean hasAllRoles = subject.hasAllRoles(Arrays.asList("role1","role2"));
System.out.println("多个角色授权:"+hasAllRoles);
//==========分隔符============
//基于资源的授权,括号内放的是权限标识符
boolean permitted = subject.isPermitted("user:create");
System.out.println("单个资源的授权:"+permitted);
//使用check方法进行授权,如果授权不通过会抛出异常
subject.checkPermission("user:update");
boolean permittedAll = subject.isPermittedAll("user:create","user:update");
System.out.println("多个资源的授权:"+permittedAll);
}shiro-permission.ini的配置文件如下:
1
2
3
4
5
6
7
8
9
10
11
12
13
14#用户
[users]
#用户zhang的密码是123456,此用户具有role1和role2两个角色
zhangsan=123456,role1,role2
wang=123,role2
#权限
[roles]
#角色role1对资源user拥有create、update权限
role1=user:create,user:update
#角色role2对资源user拥有create、delete权限
role2=user:create,user:delete
#角色role3对资源user拥有create权限
role3=user:create权限标识符号规则:资源:操作:实例(中间使用半角冒号分隔)
user:create:01 表示对用户资源的01实例进行create操作。
user:create 表示对用户资源进行create操作,相当于user:create:*,对所有用户资源实例进行create操作。
user:*:01 表示对用户资源实例01进行所有操作。
自定义Realm进行授权
在上边的程序中我们通过shiro-permission.ini配置对权限信息进行了静态配置,但是在实际开发中往往需要从数据库中获取权限信息,这时就需要自定义realm,由realm从数据库查询权限数据。realm根据用户身份查询权限数据,将权限数据返回给authorizer(授权器)。
自定义Realm如下(认证信息跟上一节的一样,这里改动的只是授权信息):
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51package com.catchu.realm;
import java.util.ArrayList;
import java.util.List;
import org.apache.shiro.authc.AuthenticationException;
import org.apache.shiro.authc.AuthenticationInfo;
import org.apache.shiro.authc.AuthenticationToken;
import org.apache.shiro.authc.SimpleAuthenticationInfo;
import org.apache.shiro.authz.AuthorizationInfo;
import org.apache.shiro.authz.SimpleAuthorizationInfo;
import org.apache.shiro.realm.AuthorizingRealm;
import org.apache.shiro.subject.PrincipalCollection;
/**
* @author 刘俊重
* @Description 自定义Realm
* @date 2017年8月1日
*/
public class CustomRealm extends AuthorizingRealm {
/**
* @Description 用于授权
* @Author 刘俊重
* @date 2017年8月1日
*/
@Override
protected AuthorizationInfo doGetAuthorizationInfo(PrincipalCollection principals) {
//从principals中获取主身份信息
//将getPrimaryPrincipal方法值强转为真实的身份信息,即在上面认证时填充到SimpleAuthenticationInfo的身份信息
String usercode = (String) principals.getPrimaryPrincipal();
//模拟从数据库中根据身份信息查询到的权限信息(实际开发中这一步是根据身份信息从数据库中查询出来的)
List<String> permissions = new ArrayList<String>();
permissions.add("user:create");//用户的创建
permissions.add("items:add");//商品添加权限
//构建授权信息,并返回
SimpleAuthorizationInfo simpleAuthorizationInfo = new SimpleAuthorizationInfo();
simpleAuthorizationInfo.addStringPermissions(permissions);
return simpleAuthorizationInfo;
}
/**
* @Description 用于认证
* @Author 刘俊重
* @date 2017年8月1日
*/
@Override
protected AuthenticationInfo doGetAuthenticationInfo(AuthenticationToken token) throws AuthenticationException {
//从token获取principal(身份信息)
String principal = (String) token.getPrincipal();
//模拟从数据库中获取到了credentials(授权信息)
String credentials = "111111";
SimpleAuthenticationInfo simpleAuthenticationInfo = new SimpleAuthenticationInfo(principal, credentials, getName());
return simpleAuthenticationInfo;
}
}单元测试代码如下:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34// 自定义realm进行资源授权测试
@Test
public void testAuthorizationCustomRealm() {
// 创建SecurityManager工厂
Factory<SecurityManager> factory = new IniSecurityManagerFactory(
"classpath:shiro-realm.ini");
// 创建SecurityManager
SecurityManager securityManager = factory.getInstance();
// 将SecurityManager设置到系统运行环境,和spring后将SecurityManager配置spring容器中,一般单例管理
SecurityUtils.setSecurityManager(securityManager);
// 创建subject
Subject subject = SecurityUtils.getSubject();
// 创建token令牌
UsernamePasswordToken token = new UsernamePasswordToken("zhangsan",
"111111");
// 执行认证
try {
subject.login(token);
} catch (AuthenticationException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
System.out.println("认证状态:" + subject.isAuthenticated());
// 认证通过后执行授权
// 基于资源的授权,调用isPermitted方法会调用CustomRealm从数据库查询正确权限数据
// isPermitted传入权限标识符,判断user:create:1是否在CustomRealm查询到权限数据之内
boolean isPermitted = subject.isPermitted("user:create:1");
System.out.println("单个权限判断" + isPermitted);
boolean isPermittedAll = subject.isPermittedAll("user:create:1",
"user:create");
System.out.println("多个权限判断" + isPermittedAll);
// 使用check方法进行授权,如果授权不通过会抛出异常
//subject.checkPermission("items:add:1");
}shiro-realm.ini的配置文件如下:
1
2
3
4
5[main]
#自定义 realm
customRealm=com.catchu.realm.CustomRealm
#将realm设置到securityManager,相当 于spring中注入
securityManager.realms=$customRealm授权流程文字描述:
- 对subject进行授权,调用方法isPermitted(”权限标识符”)
- SecurityManager执行授权,通过ModularRealmAuthorizer执行授权
- ModularRealmAuthorizer执行realm(自定义的CustomRealm)从数据库查询权限数据,调用realm的授权方法:doGetAuthorizationInfo
- realm从数据库查询权限数据,返回ModularRealmAuthorizer
- ModularRealmAuthorizer调用PermissionResolver进行权限串比对
- 如果比对后,isPermitted中”权限标识符”在realm查询到权限数据中,说明用户访问permission串有权限,否则没有权限,抛出异常。
Shiro入门—授权
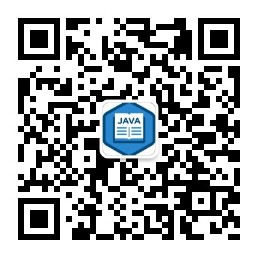
欢迎关注我的微信公众号
坚持原创技术分享